Kids With the Greatest Number of Candies
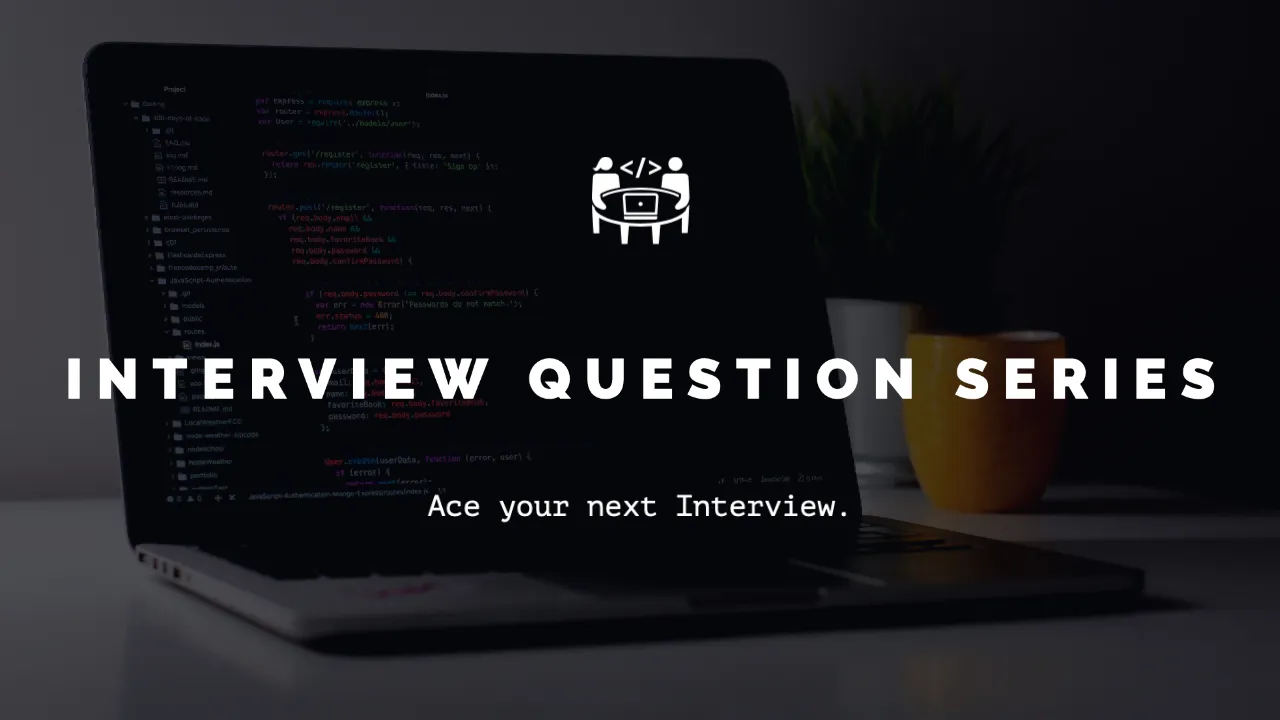
Problem Statement
Given the array candies and the integer extraCandies, where candies[i] represents the number of candies that the ith kid has.
For each kid check if there is a way to distribute extraCandies among the kids such that he or she can have the greatest number of candies among them. Notice that multiple kids can have the greatest number of candies.
Examples
Input: candies = [2,3,5,1,3], extraCandies = 3
Output: [true,true,true,false,true]
Explanation: Kid 1 has 2 candies and if he or she receives all extra candies (3) will have 5 candies --- the greatest number of candies among the kids. Kid 2 has 3 candies and if he or she receives at least 2 extra candies will have the greatest number of candies among the kids. Kid 3 has 5 candies and this is already the greatest number of candies among the kids. Kid 4 has 1 candy and even if he or she receives all extra candies will only have 4 candies. Kid 5 has 3 candies and if he or she receives at least 2 extra candies will have the greatest number of candies among the kids.
Input: candies = [4,2,1,1,2], extraCandies = 1
Output: [true,false,false,false,false]
Constraints:
2 <= candies.length <= 100
1 <= candies[i] <= 100
1 <= extraCandies <= 50
Approach
As given in the question, that we have to check if each kid can have the greatest number of candies, if any number of candy from extraCandies is being added to the current number of candies a kid is having.
The very first step is to know what is greatest in the given array. For which finding out maximum candies among all kids will work.
In second iteration, I will be adding the extraCandies to the current number of candies for each kid and check can it be same as the greatest or exceeding the greatest number.
Code
public List<Boolean> kidsWithCandies(int[] candies, int extraCandies) {
List<Boolean> res = new ArrayList();
int max = 0;
for(int i:candies){
max = Math.max(max,i);
}
for(int i:candies){
if(i+extraCandies >= max){
res.add(true);
}else{
res.add(false);
}
}
return res;
}
Complexities
Time Complexity: 2*O(n) firrst iteration to find the greatest candy. Second iteration is to find if each kid can have equal or more than the greatest candy. We can overlook the constant, the time complexity would be O(n).
Space Complexity: O(1).